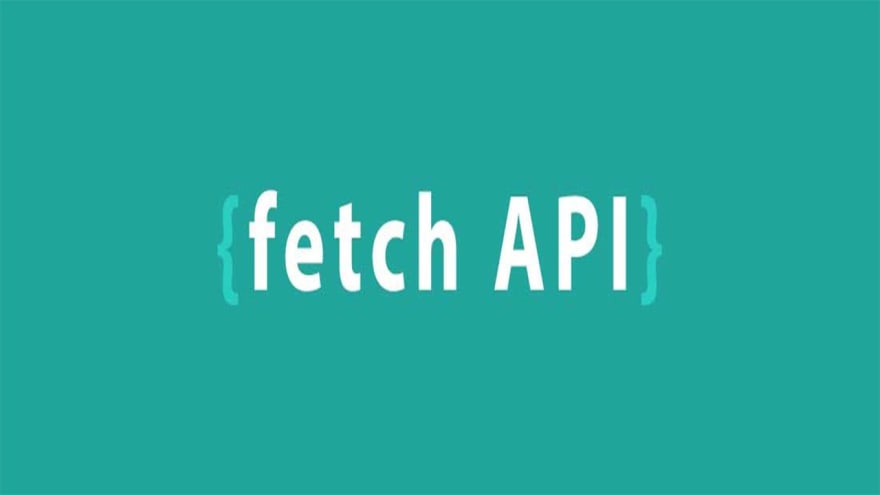
When it comes to making HTTP requests inside of JavaScript, you can use a variety of different options. For example, you can use XMLHttpRequest, a third party library like Axios, or more recently, Fetch.
What exactly is Fetch?
Fetch is a promise based HTTP request API built in to JavaScript. It’s fairly well supported (87%+) and is promise based for ease of use and is a perfect fit for those wanting to use out of the box solutions for HTTP.
Let’s take a look at a few different ways we can use fetch
to access data from the JSON Placeholder API:
GET
fetch(`https://jsonplaceholder.typicode.com/posts`)
.then(res => res.json())
.then(posts => console.log(posts))
The above fetch call has three key parts.
- We’re calling `fetch` with an API and this defaults to a GET request, returning a promise.
- This returns us a HTTP Response, and as we’re only interested in the **body** of that response, we call res.json() to extract this.
- As we now have the **data** from our API call, we can perform any action we want on it.
When it comes to other HTTP verbs such as POST/PUT/DElETE, the process is quite similar:
Customisation
Let’s use fetch to create a new post by using HTTP POST. This starts by creating the actual post:
const newPost = {
title: ‘All about Fetch!’,
body: ‘Fetch is awesome!’
}
We can now pass an options parameter into our fetch call, so let’s make that:
const options = {
method: ‘POST’,
body: JSON.stringify(newPost),
headers: new Headers({
‘Content-Type’: ‘application/json’
})
}
The options can then be passed along to our request like so:
fetch(`https://jsonplaceholder.typicode.com/posts`, options)
.then(res => res.json())
.then(posts => console.log(posts))
We can continue to customise this by adding more items to our options object, and subsequently changing the method to other values such as PUT or DELETE. Here’s an example:
const options = {
method: ‘PUT’,
cache: ‘no-cache’,
body: JSON.stringify(newPost),
headers: new Headers({
‘Content-Type’: ‘application/json’
})
}
For a full list of these and more information on how to use fetch, I recommend checking out the MDN documentation on this topic.